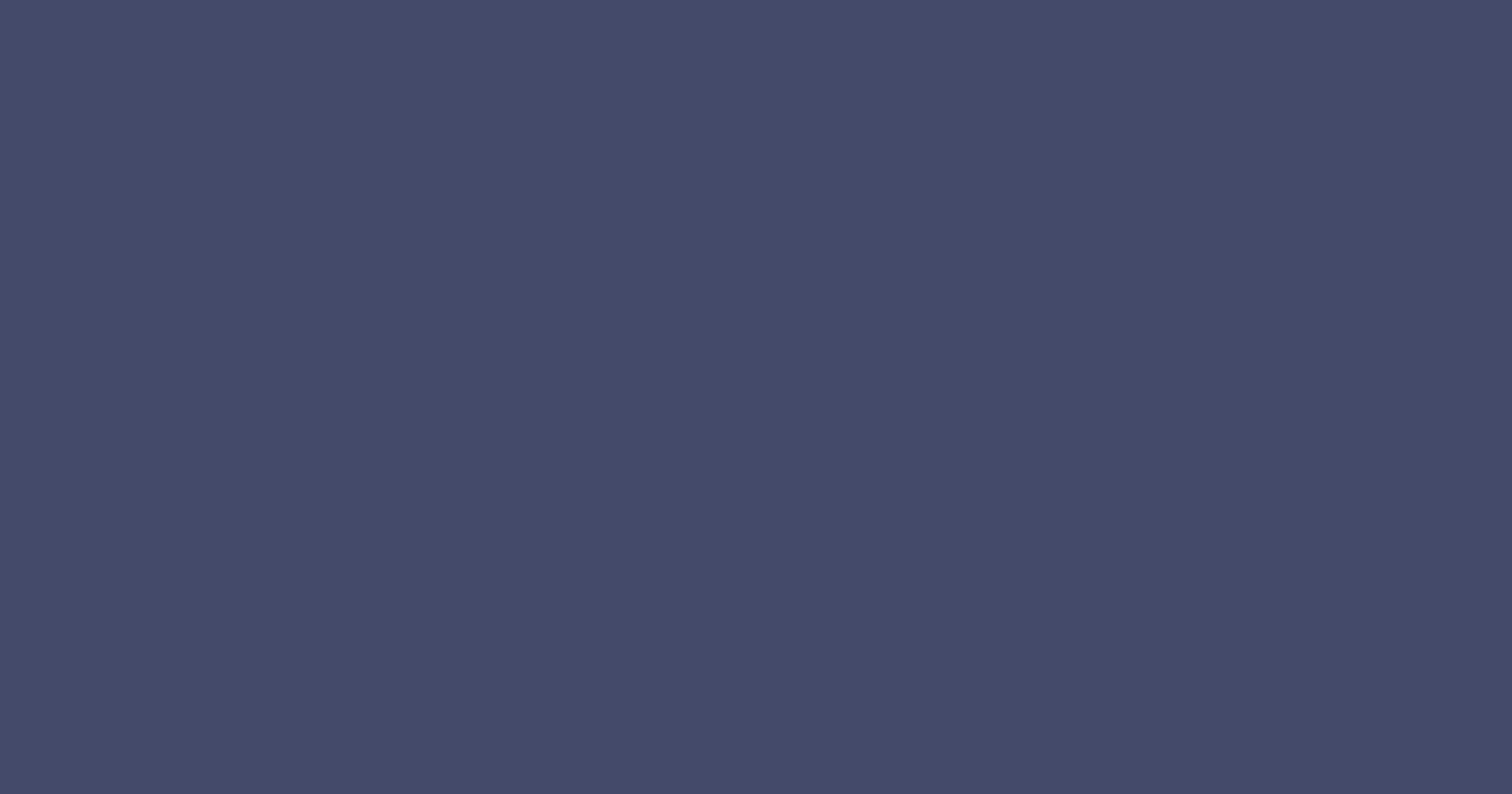
Day 3 of 30-Day .NET Challenge: Switch Constructs
The switch statements are available for creating branching logic, each offering distinct advantages based on readability and maintenance.
Introduction
The switch statements are available for creating branching logic, each offering distinct advantages based on readability and maintenance.
Learning Objectives
- Utilize the switch-case construct to compare a variable or expression with multiple potential outcomes.
Prerequisites for Developers
Utilizing the
if-else
construct to incorporate branching logic.Handling variables, employing string interpolation, and displaying output.
Getting Started
What is a switch statement?
The switch statement selects and executes a specific section of code from a list of options known as switch sections. This selection is made by matching the switch expression with predefined patterns in the switch sections.
Basic Example
switch (fruit)
{
case "apple":
Console.WriteLine($"App will display information for apple.");
break;
case "banana":
Console.WriteLine($"App will display information for banana.");
break;
case "cherry":
Console.WriteLine($"App will display information for cherry.");
break;
}
Basic Switch Example
To begin, create a static class file called “Switch.cs
” within the console application. Insert the provided code snippet into this file.
static int employeeLevel = 200;
static string employeeName = "John Smith";
/// <summary>
/// Outputs
/// John Smith, Senior Associate
/// </summary>
public static void SwitchExample()
{
string title = "";
switch (employeeLevel)
{
case 100:
title = "Junior Associate";
break;
case 200:
title = "Senior Associate";
break;
case 300:
title = "Manager";
break;
case 400:
title = "Senior Manager";
break;
default:
title = "Associate";
break;
}
Console.WriteLine($"{employeeName}, {title}");
}
Execute the code from the main method as follows
#region Day 3 - Switch Constructs
Switch.SwitchExample();
#endregion
Console Output
// Console Output
John Smith, Senior Associate
Change Switch Label
Add another method into the same static class as shown below
static int employeeLevel = 200;
static string employeeName = "John Smith";
public static void SwitchExample()
{
string title = "";
switch (employeeLevel)
{
case 100:
title = "Junior Associate";
break;
case 200:
title = "Senior Associate";
break;
case 300:
title = "Manager";
break;
case 400:
title = "Senior Manager";
break;
default:
title = "Associate";
break;
}
Console.WriteLine($"{employeeName}, {title}");
}
/// <summary>
/// John Smith, Associate
/// </summary>
public static void ChangeSwitchLabelExample()
{
employeeLevel = 201;
SwitchExample();
}
Execute the code from the main method as follows
#region Day 3 - Switch Constructs
Switch.ChangeSwitchLabelExample();
#endregion
Console Output
// Console Output
John Smith, Associate
Multiple Switch Labels
Add another method into the same static class as shown below
/// <summary>
/// Outputs
/// John Smith, Senior Associate
/// </summary>
public static void MultipleSwitchLabelExample()
{
int employeeLevel = 100;
string employeeName = "John Smith";
string title = "";
switch (employeeLevel)
{
case 100:
case 200:
title = "Senior Associate";
break;
case 300:
title = "Manager";
break;
case 400:
title = "Senior Manager";
break;
default:
title = "Associate";
break;
}
Console.WriteLine($"{employeeName}, {title}");
}
Execute the code from the main method as follows
#region Day 3 - Switch Constructs
Switch.MultipleSwitchLabelExample();
#endregion
Console Output
// Console Output
John Smith, Senior Associate
Complete Code on GitHub
GitHub — ssukhpinder/30DayChallenge.Net
Contribute to ssukhpinder/30DayChallenge.Net development by creating an account on GitHub.github.com
C# Programming🚀
Thank you for being a part of the C# community! Before you leave:
If you’ve made it this far, please show your appreciation with a clap and follow the author! 👏️️
Follow us: X | LinkedIn | Dev.to | Hashnode | Newsletter | Tumblr
Visit our other platforms: GitHub | Instagram | Tiktok | Quora
More content at C# Programming