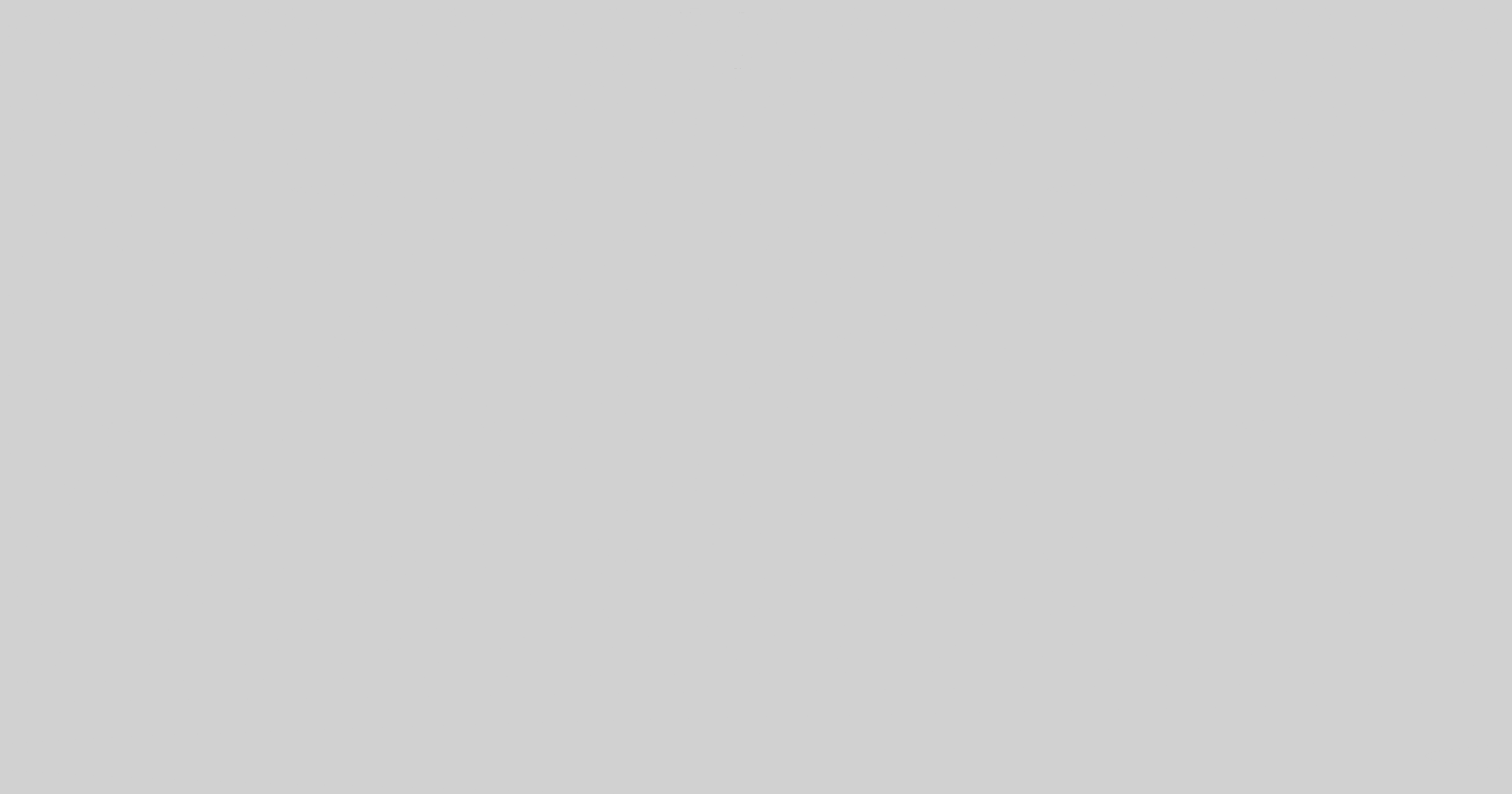
Day 1 of 30-Day .NET Challenge: Boolean Expressions
Dive in to master Boolean expressions for precise C# coding, ensuring your apps handle user data flawlessly! 🚀
Introduction
The article discusses the foundational aspects of decision logic in C# programming, focusing on Boolean expressions, operators, and their importance for developers working on C# applications dealing with customer data and user inputs.
Learning Objectives:
Use operators to construct Boolean expressions for comparison and equality testing.
Employ built-in string class methods for efficient string evaluations.
Prerequisites for Developers
Prior experience with basic coding tasks like variable instantiation, data type usage, and console output handling.
Familiarity with
if-else
constructs for conditional statements.Understanding of using the Random class for generating random numbers.
Proficiency in using Visual Studio or Visual Studio Code for creating and executing simple console applications.
Getting Started
What is an Expression?
An expression in programming combines values, operators, and methods to produce a single result. In C#, expressions are used within statements, such as “if” statements, to make decisions based on true
or false
outcomes. Boolean expressions, which return true
or false
, are pivotal for directing code execution and determining which code blocks to run. Developers select operators like equality (==)
within Boolean expressions to compare values and guide program flow based on specific conditions and logic.
Equality Operator
To begin, create a static class file called “Expressions.cs
” within the console application. Insert the provided code snippet into this file.
public static class Expressions
{
/// <summary>
/// Outputs
/// True
/// False
/// False
/// True
/// </summary>
public static void CheckEqualityOperator()
{
Console.WriteLine("a" == "a");
Console.WriteLine("a" == "A");
Console.WriteLine(1 == 2);
string myValue = "a";
Console.WriteLine(myValue == "a");
}
}
Execute the code from the main method as follows
#region Day 1 - Expressions
using _30DayChallenge.Net.Day1;
Expressions.CheckEqualityOperator();
#endregion
Console Output
// Console Output
True
False
False
True
Enhance the comparison
We can improve the comparison for string equality by utilizing the string’s inherent helper functionalities. It may seem unexpected that the line Console.WriteLine("a" == "A");
returns false. Remember, string comparisons are case-sensitive.
Let’s explore another scenario:
Console.WriteLine("a" == "a ");
In this example, a space is added at the end of one string. Consequently, this expression also yields false.
Enhance the previous equality check by applying these helper methods to both values, as demonstrated in the following code snippet:
/// <summary>
/// Outputs
/// True
/// </summary>
public static void CheckEqualityBuiltInMethods() {
string value1 = " a";
string value2 = "A ";
Console.WriteLine(value1.Trim().ToLower() == value2.Trim().ToLower());
}
Execute the code from the main method as follows
#region Day 1 - Expressions
using _30DayChallenge.Net.Day1;
Expressions.CheckEqualityBuiltInMethods();
#endregion
Console Output
// Console Output
True
Inequality Operator
The inequality operator’s result is the reverse of what the equality operator yielded.
Add another function to the Expressions.cs
class as follows
/// <summary>
/// Outputs
/// False
/// True
/// True
/// False
/// </summary>
public static void CheckInEqualityOperator()
{
Console.WriteLine("a" != "a");
Console.WriteLine("a" != "A");
Console.WriteLine(1 != 2);
string myValue = "a";
Console.WriteLine(myValue != "a");
}
Execute the code from the main method as follows
#region Day 1 - Expressions
using _30DayChallenge.Net.Day1;
Expressions.CheckInEqualityOperator();
#endregion
Console Output
// Console Output
False
True
True
False
Evaluating comparisons
For numeric comparisons, utilize operators such as:
Greater than (>)
Less than (<)
Greater than or equal to (>=)
Less than or equal to (<=)
Add another function to the Expressions.cs
class as follows
/// <summary>
/// Outputs
/// False
/// True
/// True
/// True
/// </summary>
public static void CheckComparisonOperator()
{
Console.WriteLine(1 > 2);
Console.WriteLine(1 < 2);
Console.WriteLine(1 >= 1);
Console.WriteLine(1 <= 1);
}
Execute the code from the main method as follows
#region Day 1 - Expressions
using _30DayChallenge.Net.Day1;
Expressions.CheckComparisonOperator();
#endregion
Console Output
// Console Output
False
True
True
True
Boolean return type
Certain methods in programming return a Boolean value (true
or false
). In this exercise, utilize a built-in method from the String
class to check if a larger string contains a specific word or phrase.
Add another function to the Expressions.cs
class as follows
/// <summary>
/// Check if method contains a substring
/// </summary>
public static void CheckBooleanMethods()
{
string pangram = "The quick brown fox jumps over the lazy dog.";
Console.WriteLine(pangram.Contains("fox"));
Console.WriteLine(pangram.Contains("cow"));
}
Execute the code from the main method as follows
#region Day 1 - Expressions
using _30DayChallenge.Net.Day1;
Expressions.CheckBooleanMethods();
#endregion
Console Output
// Console Output
True
False
Complete Code on GitHub
GitHub - ssukhpinder/30DayChallenge.Net